|
Using Midi Softbits, programming the Arduino to process midi data is straight forward.
// midi example using softbits
#define NCHAINS 20
// which sets of bits we want to use.
#include "softbits.h"
#include "soundbits.h"
#include "midibits.h"
// this function is only called once after a reset.
void setup() {
soft_setup();
midi_setup(0); // 0 is rxtx, 1 is USB if available
}
// snap your bits together
// this is where the MAGIC happens :)
// this function is called 1000s of times a second.
void loop() {
power_on(1);
input_midi(); // read midi data.
output_a9();
power_off();
}
|
The Midi Interface is a module that allows the LittleBits Arduino Bit to communicate with
other Midi devices such as keyboards and computers. This Midi interface was built on a
LittleBits Perf Bit.
Partslist:
- 6N138
- Opto Isolator. An opto isolator contains an LED and a photo detector. Between the
two is a gap that isolates the two parts electrically.
- Resistors
- A number of resistors. 3 x 220 ohm, 1 x 1K ohm, 1 x 10k ohm
- LittleBits Perf Bit
- The perf bits is desgined for prototyping circuits.
- 5 pin Din sockets
- 2 x 5 pin Din sockets. Midi only uses 2 of the pins.
- 8 pin IC sockets
- 1 x 8 pin IC sockets. For the 6N138.
- Optional LEDs
- 2 x LED. Used to show power and transmit activity.
- Resistors for LEDs
- 2 x 1K ohm. Used to limit the current through the LEDs.
Midi Softbits are a library of Softbits that allow the Arduino to process and generate Midi data.
More on Softbits can be found HERE
Power
Input
Output
Action
Wire
Logic
- midi_setup(int dev)
- Setup
for Midi data processing. The dev value selects a 'midi device'. 0 selects the Rx/Tx interface. 1 selects the USB
serial interface.
- input_midi()
- Read and process
Midi data from the selected devices. The Midi data bytes are decoded to messages such as note on and note off.
The messages are put into a queue. Each call to input_midi() will try and read all the available midi data before
returning. The first message is removed from the queue and put into global variables for the other midibits to use. If the message
is a note on or note off, then the value of the current chain will be set to the note value.
-
- From Softbits
-
- input_a0()
- Read the a0 analog input. Output is any value from 0 to 255.
- input_a1()
- Read the a1 analog input. Output is any value from 0 to 255.
-
- output_a5()
- Output to d5 as analog output. Output is any value from 0v to +5v.
Switch in analog position.
- output_a9()
- Output to d9 as analog output. Output is any value from 0v to +5v.
Switch in analog position.
-
- Midibits
-
- midi_gate(int send)
- The gate value is 0 if no note is playing or 255 if a note is
on. The value is sent to the specified chain's start value.
- midi_velocity(int send)
- Midi note on messages contain both the note being played and if the
'keyboard' supports it the velocity of the key. The faster (harder) the key is pressed the larger the number.
A velocity of 0 has a special meaning of note off. The value is sent to the specified chain's start value.
- midi_cc(int ctrl, int send)
- Midi supports many controllers. When a controller
is changed it sends a Control Change message. The message specifies which controller was changed and what the value is.
The ctrl parameter specifies the controller to look for and the send parameter specifies the softbits chain number to send it to.
-
- Soundbits
-
- env_attack(int rate)
- env_attack implements the
Attack part of an envelope generator. When the input is high ( greater than 128 ) then the attack bit will increase the
envelope value using the current value of the specified chain. When the envelope value hits it's maximum, the attack bit will
switch to the next bit in the generator is one is specified. If during the attack phase, the input value goes low then the attack phase
will end immediately and pass on to the next envelope bit.
- env_release(int rate)
- env_release implements the
Release part of an envelope generator. When the input is low ( less than 128 ) when activated, the release bit will decrease the
envelope value using the current value of the specified chain. When the envelope value reaches 0, the envelope cycle ends.
If during the release phase, the input value goes high then the release phase
will end immediately.
- env_value()
- env_value sets the current
chain value to the envelope value.
Source of the Softbits Midi Sketch that is loaded onto the LittleBits Arduino module. This source can be configured for
both the LittleBits Arduino 'Bit' which is an "Arduino Leonardo" board type or an "Arduino UNO" style board.
|
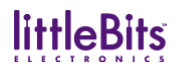
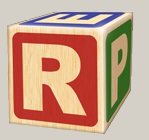
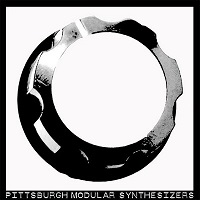
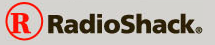
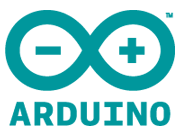
|